The first two of the phase pieces Steve Reich made in the sixties, working with recorded sounds and tape loops, were It’s Gonna Rain (1965) and Come Out (1966), both of which are made of two loops of the same fragment of speech slowly going out of phase with each other and then coming back together as the two tape players run at slightly different speeds. I was curious to see if I could make phase pieces with Sonic Pi, and it turns out it takes little code to do it.
Here is the beginning of Reich’s notes on “It’s Gonna Rain (1965)” in Writings on Music, 1965–2000 (Oxford University Press, 2002):
Late in 1964, I recorded a tape in Union Square in San Francisco of a black preacher, Brother Walter, preaching about the Flood. I was extremely impressed with the melodic quality of his speech, which seemed to be on the verge of singing. Early in 1965, I began making tape loops of his voice, which made the musical quality of his speech emerge even more strongly. This is not to say that the meaning of his words on the loop, “it’s gonna rain,” were forgotten or obliterated. The incessant repetition intensified their meaning and their melody at one and the same time.
Later:
I discovered the phasing process by accident. I had two identical tape loops of Brother Walter saying “It’s gonna rain,” and I was playing with two inexpensive tape recorders—one jack of my stereo headphones plugged into machine A, the other into machine B. I had intended to make a specific relationship: “It’s gonna” on one loop against “rain” on the other. Instead, the two machines happened to be lined up in unison and one of them gradually started to get ahead of the other. The sensation I had in my head was that the sound moved over to my left ear, down to my left shoulder, down my left arm, down my leg, out across the floor to the left, and finally began to reverberate and shake and become the sound I was looking for—“It’s gonna/It’s gonna rain/rain”—and then it started going the other way and came back together in the center of my head. When I heard that, I realized it was more interesting than any one particular relationship, because it was the process (of gradually passing through all the canonic relationships) making an entire piece, and not just a moment in time.
The audio sample
First I needed a clip of speech to use. Something with an interesting rhythm, and something I had a connection with. I looked through recordings I had on my computer and found an interview my mother, Kady MacDonald Denton, had done in 2007 on CBC Radio One after winning the Elizabeth Mrazik-Cleaver Canadian Picture Book Award for Snow.
She said something I’ve never forgotten that made me look at illustrated books in a new way:
A picture book is a unique art form. It is the two languages, the visual and the spoken, put together. It’s sort of like a—almost like a frozen theatre in a way. You open the cover of the books, the curtain goes up, the drama ensues.
I noticed something in “theatre in a way,” a bit of rhythmic displacement: there’s a fast ONE-two-three one-two-THREE rhythm.
That’s the clip to use, I decided: the length is right (1.12 seconds), the four words are a little mysterious when isolated like that, and the rhythm ought to turn into something interesting.
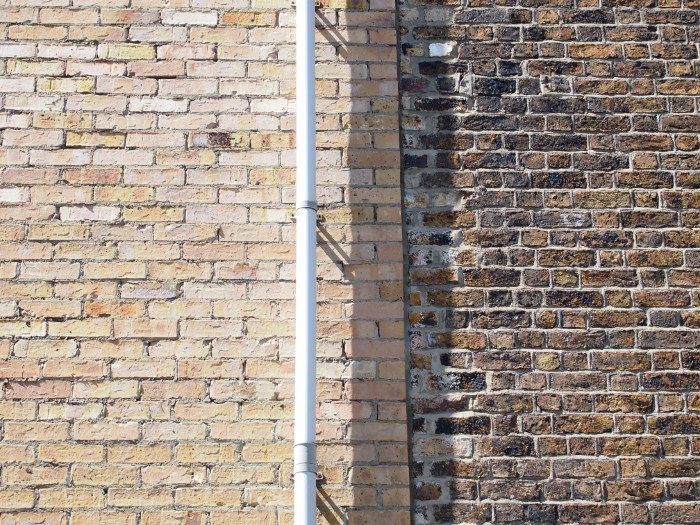
Phase by fractions
The first way I made a phase piece was not with the method Reich used but with a process simpler to code: here one clip repeats over and over while the other starts progressively later in the clip each iteration, with the missing bit from the start added on at the end.
The start
and finish
parameters specify where to clip a sample: 0 is the beginning, 1 is the end, and ratio
grows from 0 to 1. I loop n+1
times to make the loops play one last time in sync with each other.
full_quote = "~/music/sonicpi/theatre-in-a-way/frozen-theatre-full-quote.wav"
theatre_in_a_way = "~/music/sonicpi/theatre-in-a-way/frozen-theatre-theatre-in-a-way.wav"
length = sample_duration theatre_in_a_way
puts "Length: #{length}"
sample full_quote
sleep sample_duration full_quote
sleep 1
4.times do
sample theatre_in_a_way
sleep length + 0.3
end
# Moving ahead by fractions of a second
n = 100
(n+1).times do |t|
ratio = t.to_f/n # t is a Fixnum, but we need ratio to be a Float
# This one never changes
sample theatre_in_a_way, pan: -0.5
# This one progresses through
sample theatre_in_a_way, start: ratio, finish: 1, pan: 0.5
sleep length - length * ratio
sample theatre_in_a_way, start: 0, finish: ratio, pan: 0.5
sleep length*ratio
end
This is the result:
“Music as a gradual process”
A few quotes from Steve Reich’s “Music as a Gradual Process” (1968), also in Writings on Music, 1965–2000:
I do not mean the process of composition but rather pieces of music that are, literally, processes.
The distinctive thing about musical processes is that they determine all the note-to-note (sound-to-sound) details and the overall form simultaneously. (Think of a round or infinite canon.)
Although I may have the pleasure of discovering musical processes and composing the material to run through them, once the process is set up and loaded it runs by itself.
What I’m interested in is a compositional process and a sounding music that are one and the same thing.
When performing and listening to gradual musical processes, one can participate in a particular liberating and impersonal kind of ritual. Focusing in on the musical process makes possible that shift of attention away from he and she and you and me outwards toward it.
In Sonic Pi we do all this with code.
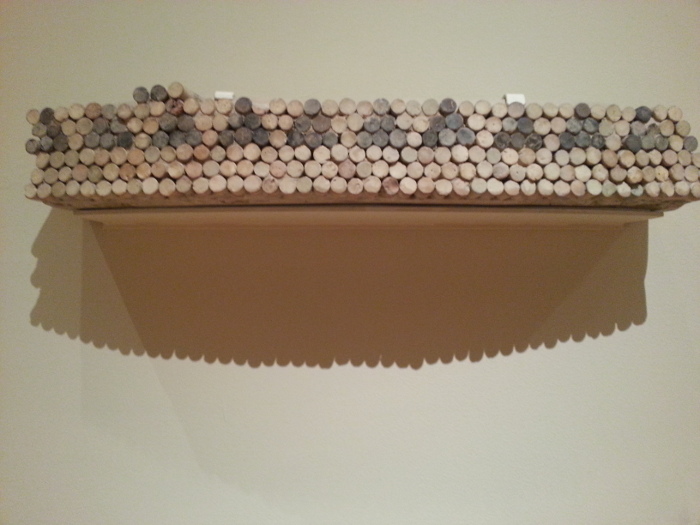
Phase by speed
The second method is to run one loop a tiny bit faster than the other and wait for it to eventually come back around and line up with the fixed loop. This is what Reich did, but here we achieve the effect with code, not analog tape players.
The rate
parameter controls how fast a sample is played (< 1 is slower, > 1 is faster), and if n
is how many times we want the fixed sample to loop then the faster sample will have length length - (length / n)
and play at rate (1 - 1/n.to_f)
(the number needs to be converted to a Float for this to work). It needs to loop n * length / phased_length)
times to end up in sync with the steady loop. (Again I add 1 to play both clips in sync at the end as they did in the beginning.)
For example, if the sample is 1 second long and n = 100, then the phased sample would play at rate 0.99, be 0.99 seconds long, and play 101 times to end up, after 100 seconds (actually 99.99, but close enough) back in sync with the steady loop, which took 100 seconds to play 1 second of sound 100 times.
It took me a bit of figuring to realize I had to convert numbers to Float or Integer here and there to make it all work, which is why to_f
and to_i
are scattered around.
full_quote = "~/music/sonicpi/theatre-in-a-way/frozen-theatre-full-quote.wav"
theatre_in_a_way = "~/music/sonicpi/theatre-in-a-way/frozen-theatre-theatre-in-a-way.wav"
length = sample_duration theatre_in_a_way
puts "Length: #{length}"
sample full_quote
sleep sample_duration full_quote
sleep 1
4.times do
sample theatre_in_a_way
sleep length + 0.3
end
# Speed phasing
n = 100
phased_length = length - (length / n)
# Steady loop
in_thread do
(n+1).times do
sample theatre_in_a_way
sleep length
end
end
# Phasing loop
((n * length / phased_length) + 1).to_i.times do
sample theatre_in_a_way, rate: (1 - 1/n.to_f)
sleep phased_length
end
This is the result:
Set n to 800 and it takes over fifteen minutes to evolve. The voice gets lost and just sounds remain.
“Time for something new”
In notes for “Clapping Music (1972)” (which I also did on Sonic Pi), Reich said:
The gradual phase shifting process extremely useful from 1965 through 1971, but I do not have any thoughts of using it again. By late 1972, it was time for something new.